This is part two of my multi-part series on my checkers web app. Part one is an introduction which explains what I was trying to do. Hopefully it's coherent, I wrote it last September and since I have quarantine head these days, I haven't gone back and revisited it.
API
My plan was to build a single page app for practice with the React JavaScript library. This type of app exists only in the browser, so it needed a server to connect to to get the data. The backend server waits for requests from the app to provide the state of the board, the players, and any other state that might be required.
APIs
An Application Programming Interface (API) is just an interface between different pieces of software, for the use of other software. So you wouldn't say a web page is an API, because it's not really written for other software to interact with. But a library that you import in a programming language provides an API to use it, and a web API also provides an interface to another system. For instance JavaScript provdes a JSON
library with an interface including JSON.parse(...)
and JSON.stringify(...)
. On the other hand, the National Weather Service provides a web API for retrieving weather data. You can see an example of using the NWS API here. (Note that that github link is to an old project that is unmaintained).
Django REST Framework
The checkers API is built using a Django web server with Django REST Framework (DRF) layered on top. DRF is a framework for building RESTful APIs on top of a standard Django web app. REST (REpresentational State Transfer) is an approach to writing APIs that focuses on transferring text in a predefined way, often over HTTP using the standard methods.
Ok, so that was a lot of jargon. Bottom line, much of the time when you write a REST API, you'll be interested in transferring objects of some sort. Typically, you'll use a GET request to get an object, a POST request to store updates an object, and a PUT request to create a new object. Often, these will all be to the same endpoint (or URL). You might use an endpoint called /thunderstorm/
to see if there were a thunderstorm in your area. Making a GET request might give you a response like this (in JSON):
{
"zip": "06268",
"thuderstorm": true,
}
Now if your API allowed you to create thunderstorms (what kind of API are you writing?) you might be able to use a PUT request to create the storm and a POST request to change the storms location.
Ok, this example is kind of getting away from me. I'll just mention three specific things that I like about DRF and link into the checkers code.
viewsets
The python Django web framework has a concept of 'views' which are functions that handle requests to endpoints in a web app. Imagine you want people to be able to contact you to request a thunderstorm on their enemies. For a contact form, reachable at /app/contact_form.html
, you might write a 'contact' view that handled both GET to render the form, and POST to handle the form submission. Somewhere in the code to handle POST you'd find something that sent your storm request to the app owner.
Viewsets are a DRF construct that provides a lot of the boilerplate views you would use to manipulate objects in a REST API. For instance, say you had a User you wanted to interact with. You might want to be able to CREATE, READ, and UPDATE a user. In DRF, your UserViewset
could give you most of that out of the box, in the way a ModelView might give you similar things in vanilla Django.
Here is the UserViewset
for checkers. It allows you to get a listing of all users (/users/
), see the details of a user (/users/3/
), and the endpoint (/users/
) also allows you to create a user using a POST request or update a user using a PUT request. There is also a special endpoint at /users/me/
that provides the user info on the logged in user.
serializers
Serializers are another DRF feature analogous to Django templates. If you've ever used a templating system (jinja, handlebars, or many, many others) for generating your webpage, you can think of something like that. Serializers are designed for outputting the kind of representations that APIs use, such as JSON or XML, instead of HTML like a web template might create.
I'll link to the checkers user serializer. It's a super simple mostly generic serializer, but it provides enough to allow get and post requests. There's no validation done here, besides what is provided by the framework.
the http interface
Django Rest Framework provides a web frontend to talk to the API. If I had a live checkers instance, I'd point to that, but at the time of this writing, it doesn't exist. Here's a taste, though:
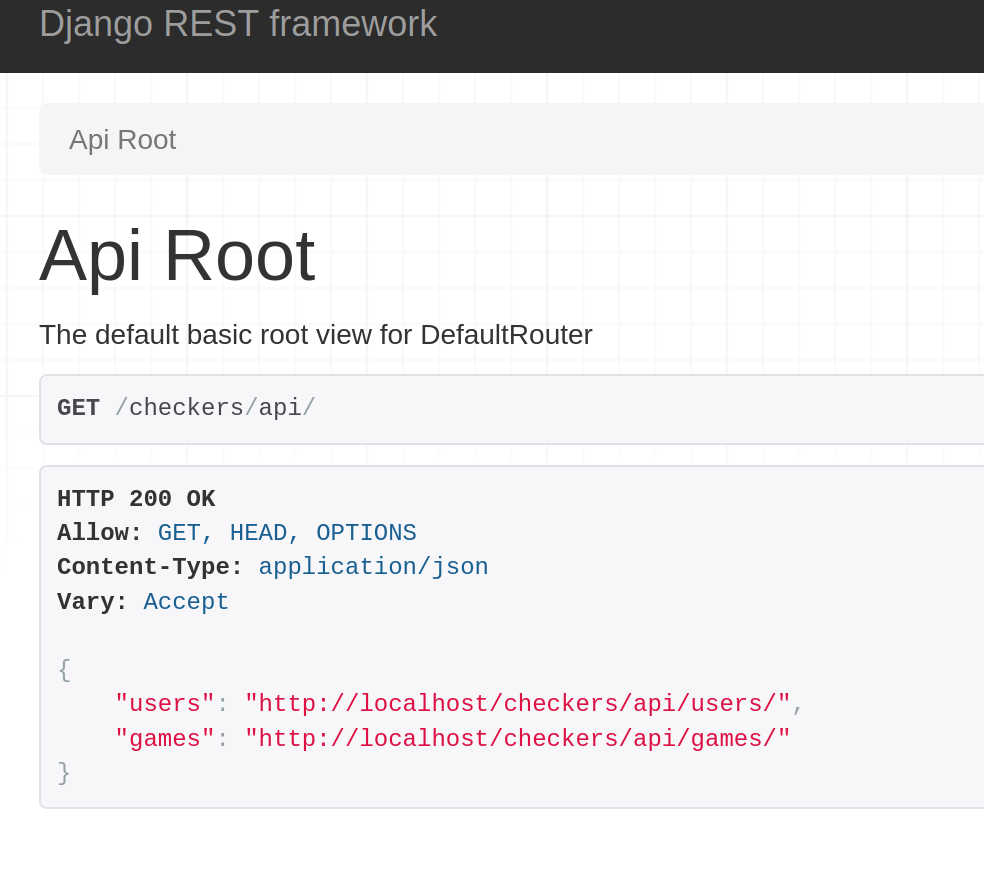
This is incredibly helpful as it allows you to browse and test the API as you would click around on a normal website. It allows you to see exactly what data your serialziers are providing, as well as access the various endpoints and submit and retrieve data.
Checkers Endpoints
So with the libraries out of the way, lets look at the various checkers end points. There aren't many, since the viewsets cover most of the useful views. users
allows you to get and set user information. game
provides information about the game, and provides additional endpoints for move
and jump
to make moves. board
still exists but is vestigial, because I now get the board information through the game endpoint.
Coming Up
Hopefully this provides enough background to talk about some interesting react things next time. Next time I'll talk about React and JavaScript. My plan is to break it up and talk about a few interesting things at a time.
Until next time, fair winds!
cover: Photo by Artem Labunsky on Unsplash